As we know, Active Storage analyzes files once they have been uploaded.
The result of this is a metadata hash that is stored in the metadata column in the active_storage_blobs
table.
Until Rails 7, the metadata for video files held the following values -
width
, height
, duration
, angle
, display_aspect_ratio
.
With a recent PR to
Rails,
the metadata hash will now also contain an audio value of type boolean
to represent the existence of an audio channel in the video file.
Before
Let’s say we have a Post
model that has_one_attached :video
-
=> Post.first.video.metadata
=> {"identified"=>true, "width"=>1920.0, "height"=>1080.0, "duration"=>4.688017, "display_aspect_ratio"=>[16, 9], "analyzed"=>true}
Notice how we don’t have an audio
value in our metadata hash.
After
Considering the same scenario above, in Rails 7, we get the following -
=> Post.first.video.metadata
=> {"identified"=>true, "width"=>1920.0, "height"=>1080.0, "duration"=>4.688017, "display_aspect_ratio"=>[16, 9], "audio"=>false, "video"=>true, "analyzed"=>true}
This metadata hash contains an audio value that is of type boolean
and represents whether the file has an audio channel present.
Now let’s see how we can use this new addition in our example app.
Let’s,
say that we want to add a validation constraint to check to see if an audio channel exists.
In our Post
model,
# app/models/post.rb
class Post < ApplicationRecord
has_one_attached :video
validate :acceptable_video
def acceptable_video
# Only run our validation if a video is attached
return unless video.attached?
unless video.metadata[:audio]
errors.add(:video, "requires audio to be a valid upload")
end
end
end
Trying to add a new post with a video file with no audio will give you an error like so -
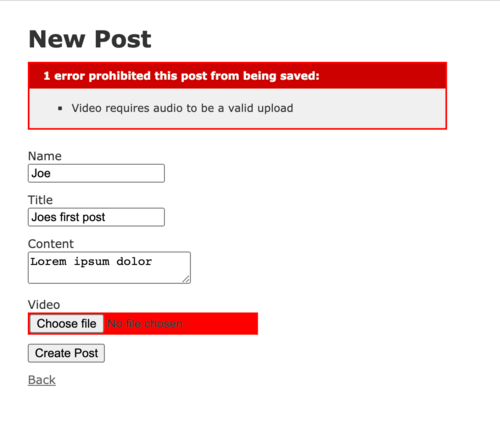