In today’s information-driven world, it is essential for every business to understand its customers’ needs and demands. One of the best ways to do this is to monitor customers’ interactions with our website through Google Analytics.
Fortunately, Google has simplified this process by enabling its integration with our React application. As a developer, this interaction will favor us in many ways. It will allow us to track any bug in our application, optimize the application based on the user’s interests and behavior data, and more.
In this blog, we will explain the entire process of integrating Google Analytics Tracking Code in our React Js using a few simple steps.
Step 1: Create a Property in Google Analytics
Navigate to analytics.google.com and create a new account.
Next, we have to create a new property for our website. This allows Google to track our website. To do this-
- First, go to the dashboard and click on
Admin
(check at bottom-left corner) »Create New Property
- Now, fill in the property name eg. Blog
- Click on
Show advanced options
- Turn on the
Create a Universal Analytics property
option - Enter the Website URL where tracking needs to be added.
We have mapped
localhost:3000
tohttps://993a-182-68-54-162.ngrok.io
using ngrok - Click on ‘Create a Universal Analytics property only’
- Click the Next button
- Now, click Create button
- Copy the
TRACKING ID
(‘UA-XXXXX-X’ in our case )
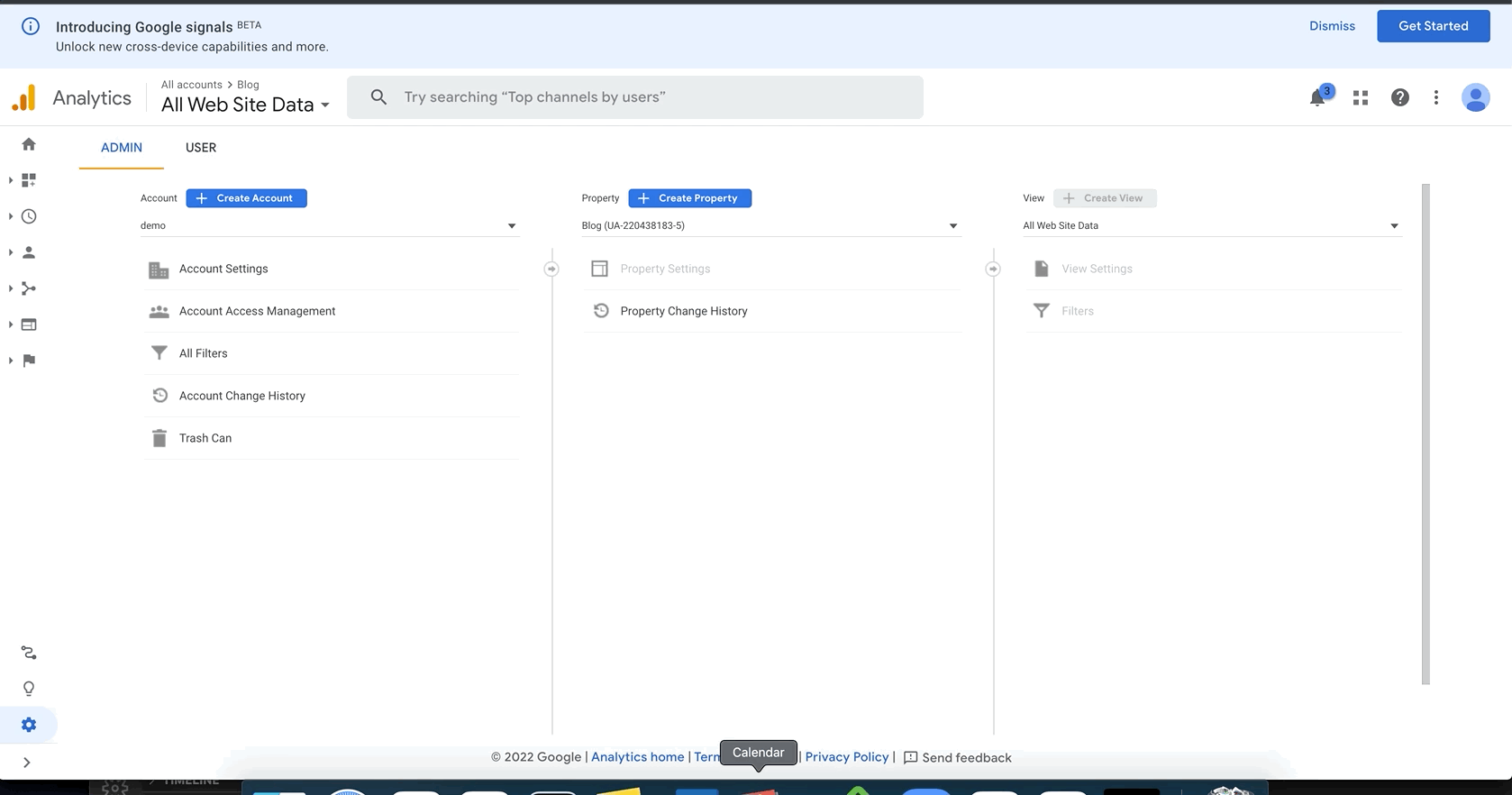
Step 2: Install Dependencies
First, we need to install the
react-ga package
from npm
.
This is the official npm
package required
for integrating our React app with
Google analytics.
yarn add react-ga
Or
npm i react-ga
Step 3: Setup Google Analytics Inside Project
To set up Google Analytics inside our project, GA needs to be initialized. This needs to be done before any of the other tracking functions will record any data.
//App.js
import ReactGA from 'react-ga';
const TRACKING_ID = "UA-XXXXX-X"; // OUR_TRACKING_ID
ReactGA.initialize(TRACKING_ID);
Step 4: Implementation
Once, Google Analytics is set within the project, we can take multiple actions like tracking how many times a user clicked on a button or filled up a form, etc. For example, if we want to how many times a user clicked on the ‘Call Us’ or ‘Write to us’ link in the contact us section, we can add GA events as below -
//useAnalyticsEventTracker.jsx
import React from "react";
import ReactGA from "react-ga";
const useAnalyticsEventTracker = (category="Blog category") => {
const eventTracker = (action = "test action", label = "test label") => {
ReactGA.event({category, action, label});
}
return eventTracker;
}
export default useAnalyticsEventTracker;
//ContactUs.jsx
import useAnalyticsEventTracker from './useAnalyticsEventTracker';
const ContactUs = () => {
const gaEventTracker = useAnalyticsEventTracker('Contact us');
return(
<div>
<h3>Contact Us</h3>
<div>
<a href="#" onClick={()=>gaEventTracker('call')}>Call Us</a>
</div>
<div>
<a href="mailto:[email protected]" onClick={()=>gaEventTracker('email')}>Write to us</a>
</div>
</div>)
};
To see if the events are getting tracked, click on Realtime » Events in the left navigation of GA.
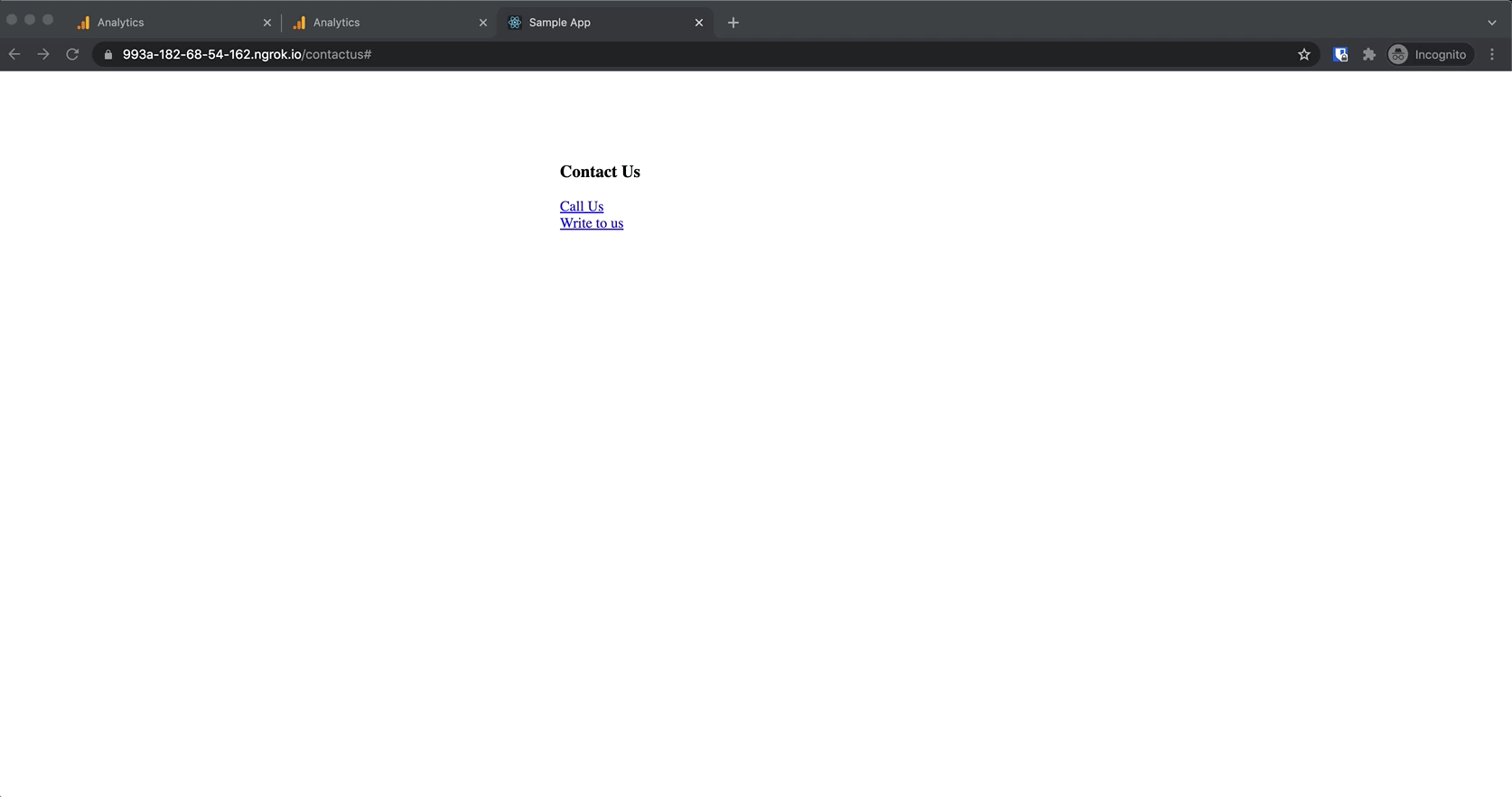
Step 5: Integration With React Router
At times, we also want to track the pages that are visited the most. However, it’s difficult to track with a CSR (Client Side Rendering) a library like React as it can load only one HTML file.
The good news is that
we have worked a way out for that.
We can leverage the react-router-dom
library to monitor the URL change.
This way, we can transmit the URL change data to Google Analytics and monitor the pages that is the most visited by users.
import React, {useEffect } from 'react';
import ReactDOM from 'react-dom';
import ReactGA from 'react-ga';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
const TRACKING_ID = "UA-220438183-5"; // OUR_TRACKING_ID
ReactGA.initialize(TRACKING_ID);
const ContactUs = () => (
<div>
<h3>Contact Us</h3>
</div>
);
const Home = () => (
<div>
<h2>Home</h2>
</div>
);
const About = () => (
<div>
<h2>About</h2>
</div>
);
const App = () => {
useEffect(() => {
ReactGA.pageview(window.location.pathname + window.location.search);
}, []);
return (
<Router>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/contactus" element={<ContactUs />} />
</Routes>
</Router>
);
}
export default App;
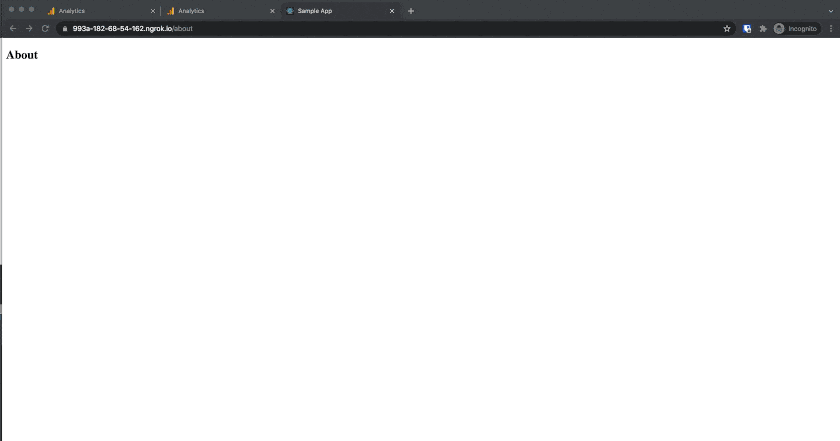
Voila! We have successfully integrated our React Application to Google Analytics and now we can easily track all the user interactions.
To track other data, check out more details here and demo here.