Many programming languages like Python, Ruby, etc
have the ability to do ‘negative indexing’
arr[-1]
.
It means
we can access the elements of the array from the end
by specifying the negative index.
Unfortunately, this feature was missing in JavaScript.
The JavaScript design made it difficult to support this feature.
The []
syntax is used for accessing array and object.
obj[1]
refers to accessing the value of key 1
.
Similarly, obj[-1]
refers to accessing the value of key -1
,
instead of returning the last property of an object.
Many approaches(accessing the .last
property)
were taken to work around this problem.
Finally, the at()
method was introduced in ECMAScript 2022
and the long-standing request from programmers was answered.
Suppose we have an array sorted in ascending order. We want to get the greatest number in the sorted array.
const sortedArray = [4, 10, 30, 100, 202, 400];
Before
- One way to get the greatest number is by accessing
the
array.length
property.
console.log(sortedArray[sortedArray.length - 1]); //400
Similarly, we can get the ‘N’th greatest element as below -
console.log(sortedArray[sortedArray.length - N]);
Using this method adds some inconvenience -
- We need to store the array in a variable.
- We need to use the array name repeatedly
- It is hostile to anonymous values.
-
If the array name is large, syntax affects the readability.
-
The other way is to use
array.slice
..slice()
already supports negative indexes so it matches our requirement.
//Greatest element
console.log(sortedArray.slice(-1)[0]); //400
//To get the 'N'th element.
console.log(sortedArray.slice(-N)[0]);
This method avoids some of the drawbacks of using length
-
- Avoids name repetition.
- Friendly to anonymous values.
However, the syntax of using array.slice
is a little weird, especially [0]
.
After
ECMAScript 2022 adds at()
method in Array, String, and TypedArray.
Syntax
at(index);
Parameter: index
The index of the array element which needs be be accessed. The ‘index’ parameter supports relative indexing from the end of the array when passed a negative index.
Return value:
The element in the array that matches the given index.
It returns undefined
if the index is not found.
// Largest element in array
console.log(sortedArray.at(-1)); //400
// 'N'th largest element.
console.log(sortedArray.at(-N));
//Undefined when index not found
console.log(sortedArray.at(100)); //undefined
//Works with string
"Saeloun".at(-1); //n
Browser compatibility
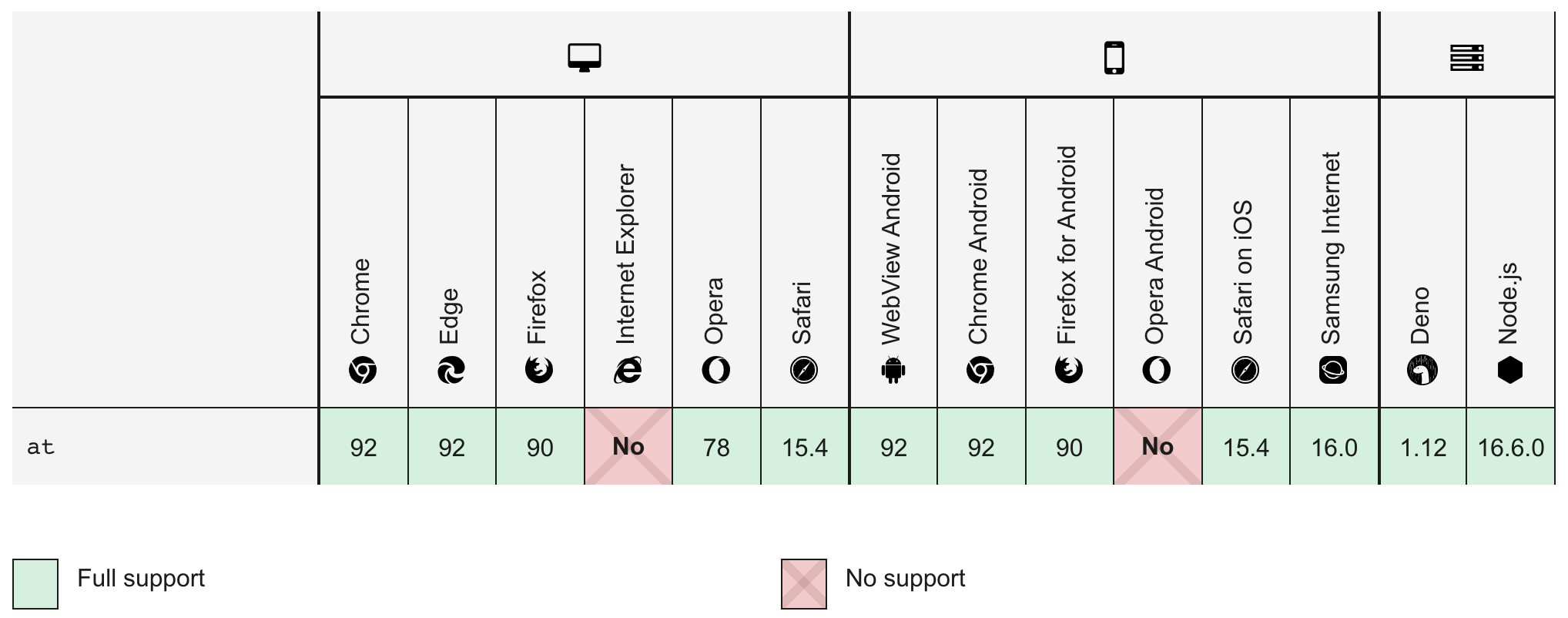
To know more about the details check out the TC39 at() proposal.