ECMAScript 2022 adds private fields, methods, and, accessors to classes. Check out our previous blog to know more.
When we access a public field on an object
that has not been declared,
we get undefined
.
However, when we try to access a private field
on an object that does not have it installed
an exception is thrown.
Sometimes, it is desirable to check if an object has a given private field, and if not, have some fallback behavior.
Before
We can use try-catch to check for the existence of a private field.
class User {
#phone = 999999999;
static phoneNumber(obj) {
//Checks if #phone field is present in obj
if (!obj.#phone) {
throw new Error(`Error!`);
}
return `Phone number :: ${obj.#phone}`;
}
}
try {
const phoneNo = User.phoneNumber(new User());
console.log(phoneNo); // Phone number :: 999999999
const newPhoneNo = User.phoneNumber({});
console.log(newPhoneNo); //Not applicable
} catch {
console.log("Not applicable");
}
Isn’t it too much to write for a simple condition?
This code also doesn’t work reliably in the presence of private getters, which may throw an exception for different reasons.
After
The in operator allows us to check whether the given object has the given property.
const user = {'phone': 999999};
console.log('phone' in user); // true
const newUser = {};
console.log('phone' in newUser); // false
The ergonomic brand check feature in ECMAScript 2022
extends the in
operator to support private class fields.
It provides a compact way for checking if an object has a private field.
class User {
#phone = 999999999;
static phoneNumber(obj) {
//Checks if #phone field is present in obj
if (!(#phone in obj)) {
return `Not applicable`;
}
return `Phone number :: ${obj.#phone}`;
}
}
const phoneNo = User.phoneNumber(new User());
console.log(phoneNo); //Phone number :: 999999999
const newPhoneNo = User.phoneNumber({});
console.log(newPhoneNo); //Not applicable
The code looks so clean now!
Browser support
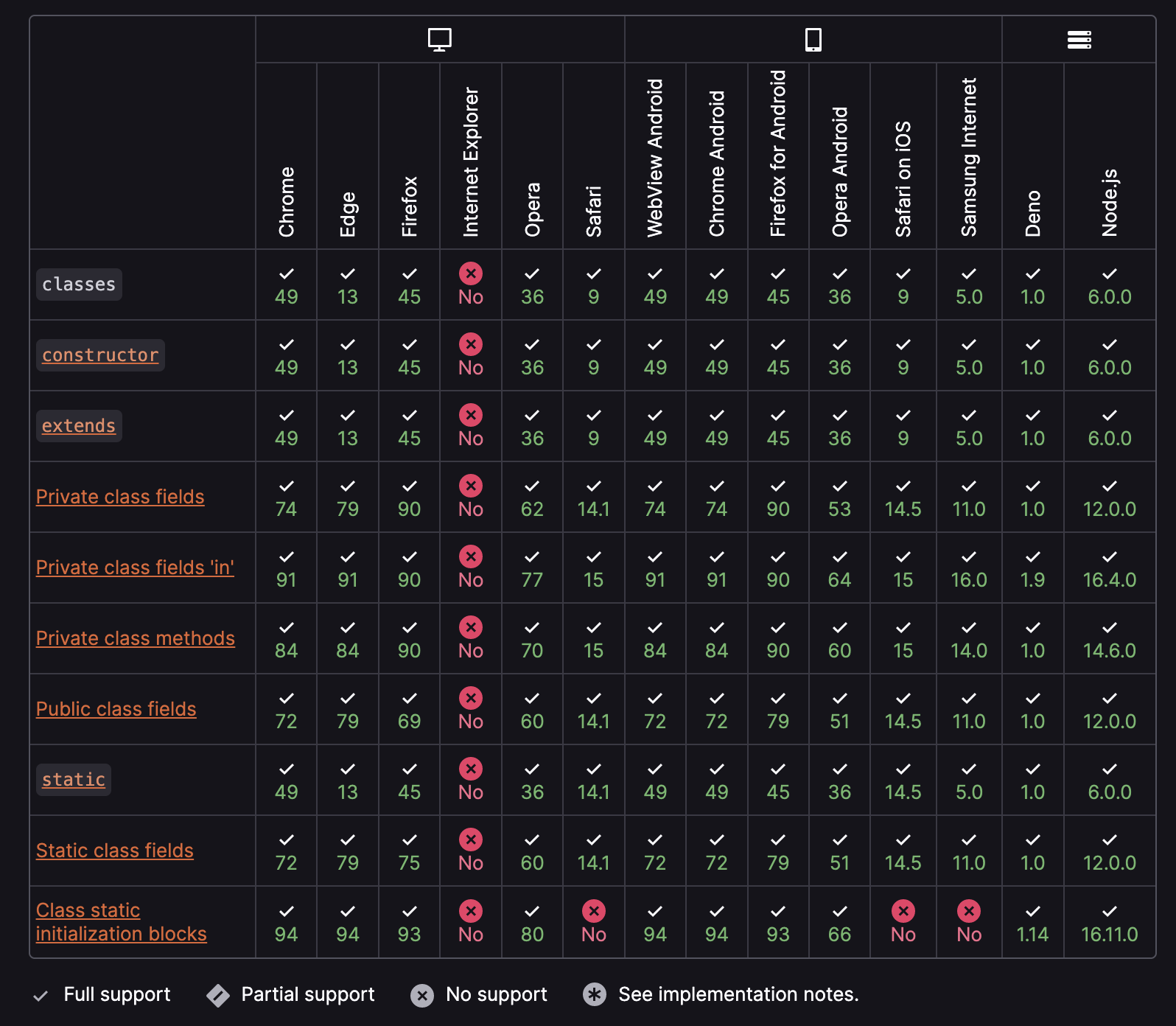
To know more about the details, check out the TC39 private fields ‘in’ operator proposal.