An important aspect of website building is being able to copy any text to the clipboard, where the user doesn’t need to select or hit the key combination on their keyboard. The entire technique can be simplified into a button that causes the code to work!
This feature is super helpful in cases where we need to copy code snippets, activation key, token, verification code, etc.
Browsers have used the
document.execCommand()
command to work with the clipboard
for a few years now.
Though it was widely used, it does not work well with large text. Being synchronous, it blocks the page giving a bad user experience. There are also permissions issues that vary depending on the browsers.
The Async Clipboard API solves these problems.
In this blog, we will look into a way to copy text and images to the clipboard using the Clipboard API.
The Asynchronous Clipboard API
The Clipboard API provides the ability to respond to clipboard commands (cut, copy, and paste) as well as to asynchronously read from and write to the system clipboard.
Clipboard Permissions
Accessing the clipboard raises several security concerns. The Clipboard API is made secure to avoid security risks.
- The Clipboard API is only supported for pages served over HTTPS.
- Clipboard access is only granted when the page is the active browser tab
- Reading from the clipboard always requires permission.
Below are the two permission associated with the Clipboard API
-
clipboard-write
permission: Controls access to the write method. -
clipboard-read
permission: Controls access to the read method.
Based on how we are using the Clipboard API,
developers always need to check for the browser’s permission,
before they try any operation.
To verify if we have the write access,
we need to use the navigator.permissions
and query for the clipboard-write
permission:
navigator.permissions.query({ name: "write-on-clipboard" }).then((result) => {
if (result.state == "granted" || result.state == "prompt") {
alert("Write access granted!");
}
});
We can also verify the clipboard-read
access
using the following code:
navigator.permissions.query({ name: "read-text-on-clipboard" }).then((result) => {
if (result.state == "granted" || result.state == "prompt") {
alert("Read access granted!");
}
});
Copying Text to Clipboard
Now, let’s go over the process of copying text to the clipboard.
To copy text with the new Clipboard API,
we have to use writeText()
method
that will accept only a single parameter, i.e. copying text to the clipboard.
<button class="btn" onclick="copyContent()">Copy!</button>
async function copyContent() {
try {
await navigator.clipboard.writeText('Content to Copy');
console.log('Content copied to clipboard');
} catch (err) {
console.error('Failed to copy: ', err);
}
}
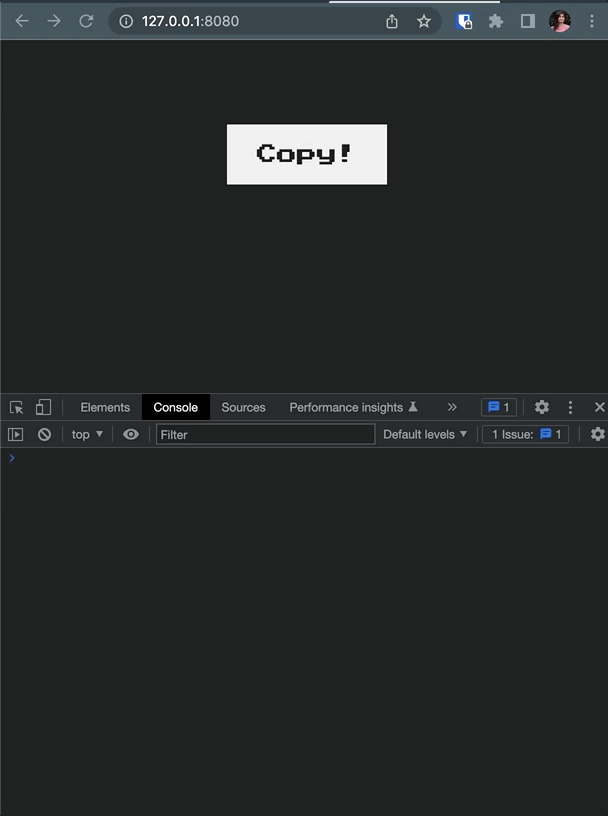
Copy Image to Clipboard
We have to use the Clipboard API’s write()
method to copy images,
and just like the previous function,
even this function accepts a single parameter -
an array containing data to be copied to the clipboard.
For example, we can extract a picture from any remote URL and copy it to the clipboard using the following code:
<button class="btn" onclick="copyPicture()">Copy Picture!</button>
async function copyPicture() {
try {
const response = await fetch('./logo.png');
const blob = await response.blob();
await navigator.clipboard.write([
new ClipboardItem({
[blob.type]: blob
})
]);
console.log('Image copied.');
} catch (err) {
console.error(err.name, err.message);
}
};
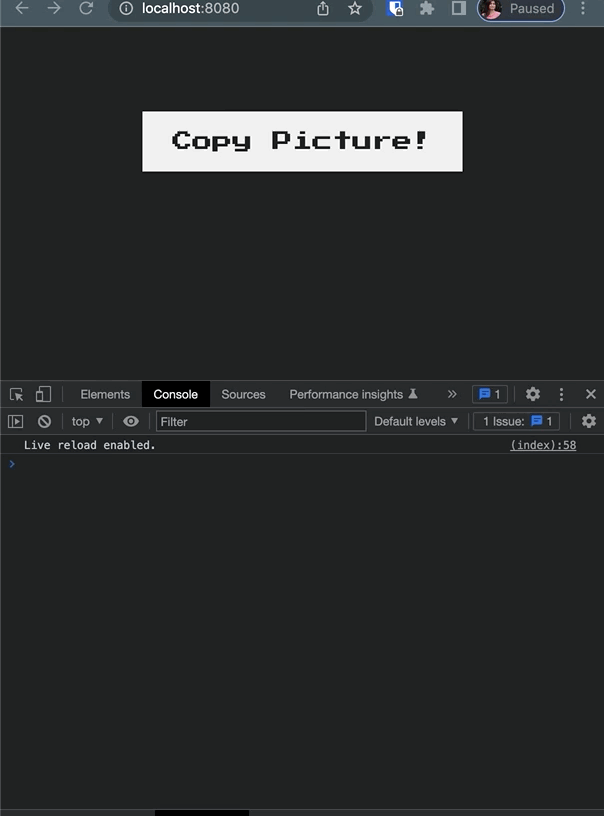
Note:
-
The
writeText()
method is meant for plain text, whilewrite()
can be used to copy any arbitrary data such as images. -
The Clipboard API may not be fully implemented to the specification in all browsers. Check out the browser compatibility before proceeding further.
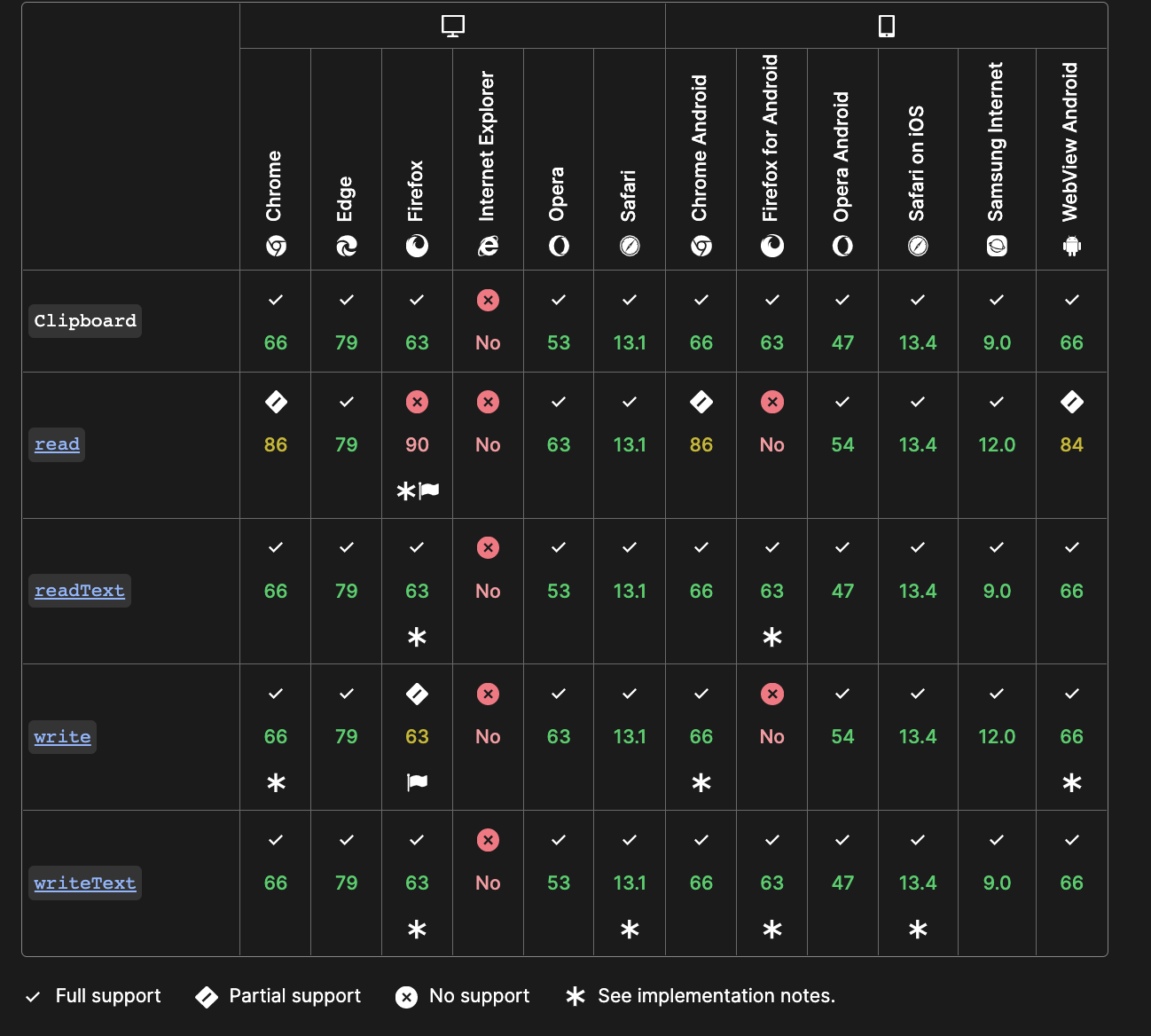
We hope this guide for copying texts, and images using the new Clipboard API was helpful.