SyntheticEvent in React is a cross-browser wrapper similar to browers’s native events;
It has the same interface as the browser’s native event,
including stopPropagation()
and preventDefault()
,
except the events work identically across all browsers.
Until React 18,
React’s synthetic media events contain several existing media events,
for instance onLoadedMetadata
and onVolumeChange
.
But there is no onResize
handler.
Before
<video>
element with a onResize prop would display a warning
Warning: Unknown event handler property `onResize`. It will be ignored.
After
With the changes
in React 18,
onResize props are added to <video>
element that triggers when one or both of the videoWidth
and videoHeight
attributes have just been updated.
It’s useful for responding to resolution changes in video players.
Sample Code
import { useState } from "react";
import "./styles.css";
const VIDEO_LINKS = [
"https://www.sample-videos.com/video123/mp4/240/big_buck_bunny_240p_2mb.mp4",
"https://www.sample-videos.com/video123/mp4/480/big_buck_bunny_480p_2mb.mp4",
"https://www.sample-videos.com/video123/mp4/720/big_buck_bunny_720p_20mb.mp4"
];
export default function App() {
const [switchVideo, setSwitchVideo] = useState(0);
const [videoWidth, setVideoWidth] = useState(null);
const [videoHeight, setVideoHeight] = useState(null);
return (
<div className="App">
<video
src={VIDEO_LINKS[switchVideo]}
controls
style={{ width: videoWidth, height: videoHeight }}
onResize={(e) => {
setVideoWidth(e.target.videoWidth);
setVideoHeight(e.target.videoHeight);
}}
/>
<div className="resButton">
<button onClick={() => setSwitchVideo(0)}>240p</button>
<button onClick={() => setSwitchVideo(1)}>480p</button>
<button onClick={() => setSwitchVideo(2)}>720p</button>
</div>
</div>
);
}
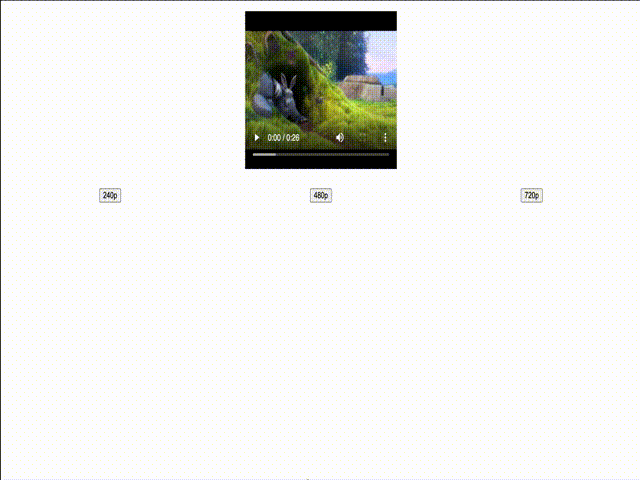